Custom Objects with Web Services
Web Services are not .net specific. Most of them might think its something Microsoft invented. What Microsoft have done is they implemented a common standard. To create a web service in .net is really easy. But you should understand the concept behind it. You can find the public web services which available through Microsoft UDDI service.(http://uddi.microsoft.com/visualstudio/ )
So today im going to explain how to return a custom object through web service. I will explain the scenario from a simple diagram.( banks needs a valid telephone number to issue a credit card its a fact)
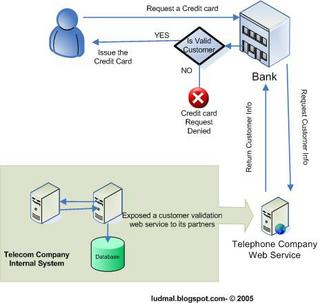
In this scenario Telephone Company is offering a web service to its partners to get the customer just by supplying customers telephone number.
Customer class:
public class Customer
{
public Customer()
{
//
// TODO: Add constructor logic here
//
}
string _name;
string _age;
string _company;
public string Name
{
get{return _name;}
set{_name = value;}
}
public string Age
{
get{return _age;}
set{_age = value;}
}
public string Company
{
get{return _company;}
set{_company = value;}
}
}
GetCustomer web service method which returns the customer object
[WebMethod]
public Customer GetCustomer()
{
//can be perform any database functions
Customer customer = new Customer();
customer.Name = "Ludmal de silva";
customer.Age = "25";
customer.Company = "E-Solutions";
return customer;
}
Now we can use this web service by simply adding a web reference to our project.(you guys should know how to add a web reference its really simple).
Finally you can get the customer object which returns from the web service. Code below.
//Customer object
CustomerService.Customer customer = new CustomerService.Customer();
//CustomerService object
CustomerService.Service1 myCustomerService = new CustomerService.Service1();
///Get the customer from the WebService
customer = myCustomerService.GetCustomer();
//Assigning the values to the UI
this.Label1.Text = customer.Name;
this.Label2.Text = customer.Company ;
this.Label3.Text = customer.Age ;
What I was trying to do is to give a basic idea about web services & to use the custom object with web services. I highly appreciate comments. (my writing skills might be poor...) But all the questions /comments are welcome.